January 11, 2022
Lists and group_by (Ruby)
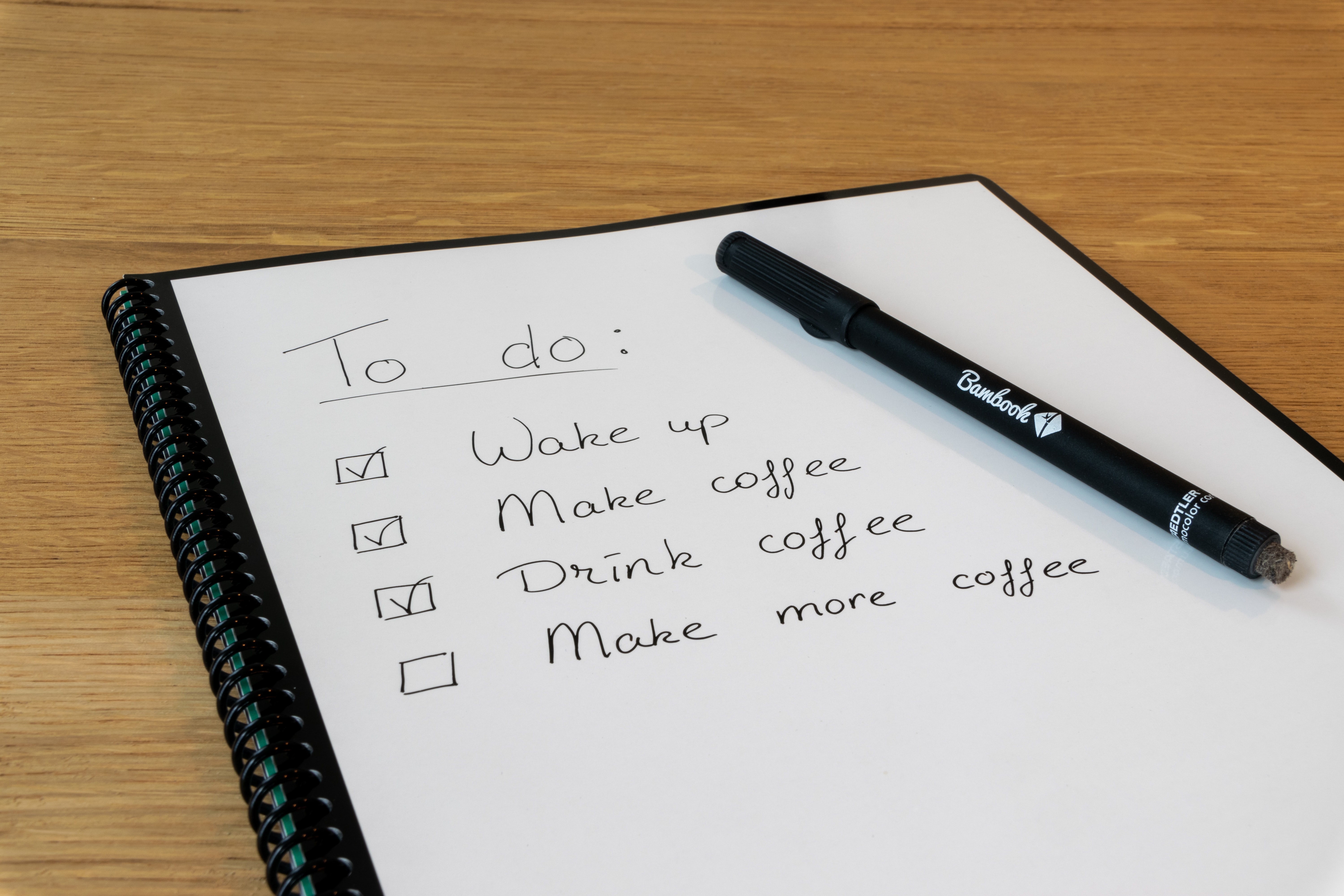
The method group_by is a very useful and easy feature for working with lists - https://apidock.com/ruby/Enumerable/group_by
For the examples, we use this simple list of cities.
cities = [ { id: 1, description: 'Porto Alegre', country: 'Brazil' }, { id: 2, description: 'London', country: 'UK' }, { id: 3, description: 'New York', country: 'USA' }, { id: 3, description: 'Rio de Janeiro', country: 'Brazil' } ]
Example 1 - Hash of objects indexed by attribute
cities.group_by{ |city| city[:id] }.transform_values(&:first)
Result
{ 1 => {id: 1, description: 'Porto Alegre', country: 'Brazil'}, 2 => {id: 2, description: 'London', country: 'UK'}, 3 => {id: 3, description: 'New York', country: 'USA'} }
Example 2 - Aggregate and counting
cities.group_by{ |city| city[:country] }.transform_values(&:count)
Result
{ 'Brazil' => 2, 'UK'=>1, 'USA'=>1 }