May 09, 2022
Manage Docker with HTTP API
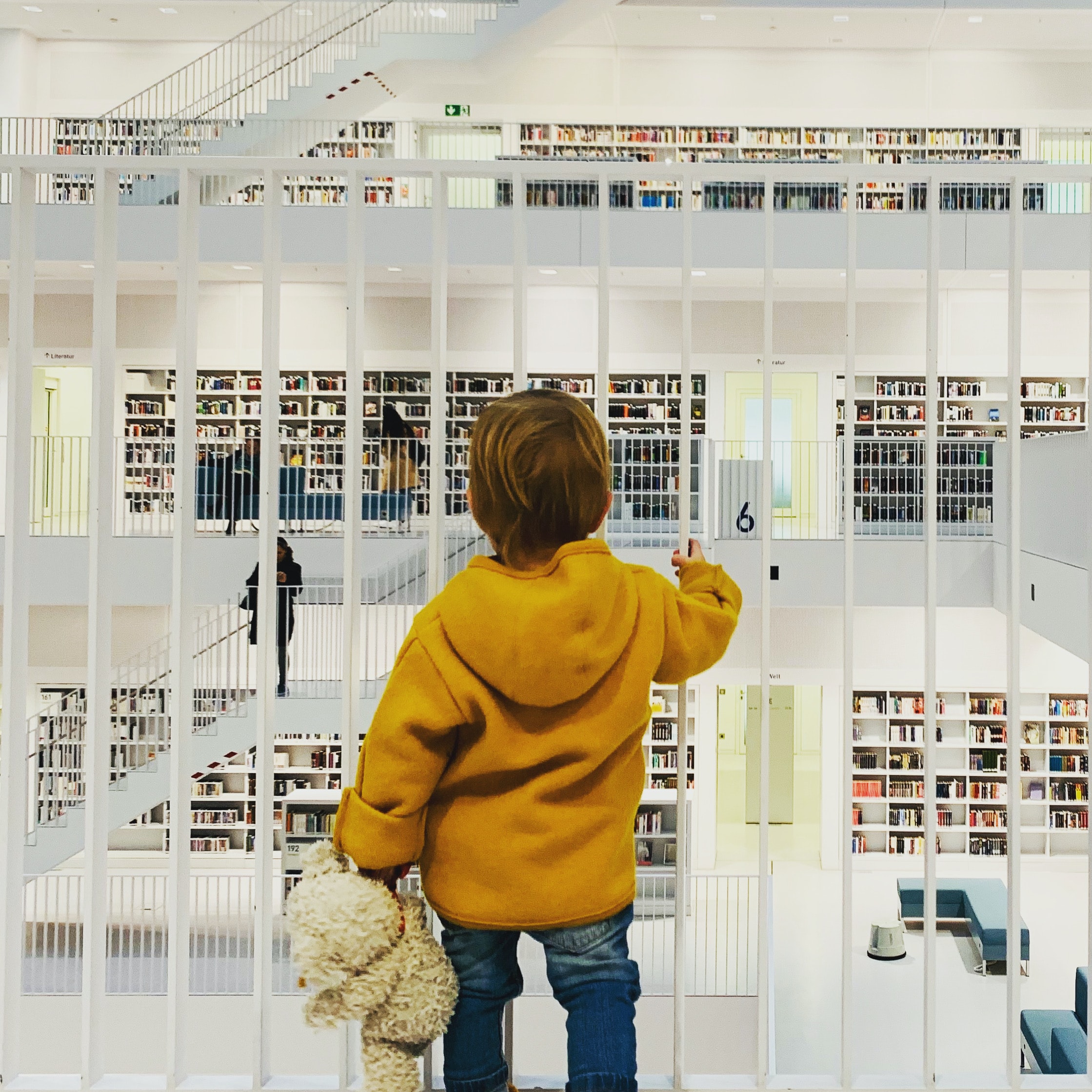
Today I'm going to talk about one of the good features of docker that's not too popular, this is the ... .... π₯π₯π₯ ... ... HTTP Docker API - https://docs.docker.com/engine/api. Yes! Docker provides an HTTP API for interacting with the Docker daemon.
The concept is simple and powerful, docker has an HTTP API that allows you to do many things, like: create containers, pull images, run containers, show active containers, etc.
For example, if you want to create a container, you can do so with the endpoint Container Create. But if you need to list current containers, you can do that with the endpoint Container List. Anyway, there are many endpoints and you can find them all in the documentation.
The first thing you need to do is enable the API, there are different steps depending on your operating system, I won't go into detail on how you can do this. For our tests we will use the local socket "unix:///var/run/docker.sock".
There isΒ a nice gem for ruby to abstract the endpoints: Docker API - A lightweight Ruby client for the Docker Remote API
To understand how you can interact with the API I built a short example, in this example I will:
The concept is simple and powerful, docker has an HTTP API that allows you to do many things, like: create containers, pull images, run containers, show active containers, etc.
For example, if you want to create a container, you can do so with the endpoint Container Create. But if you need to list current containers, you can do that with the endpoint Container List. Anyway, there are many endpoints and you can find them all in the documentation.
The first thing you need to do is enable the API, there are different steps depending on your operating system, I won't go into detail on how you can do this. For our tests we will use the local socket "unix:///var/run/docker.sock".
There isΒ a nice gem for ruby to abstract the endpoints: Docker API - A lightweight Ruby client for the Docker Remote API
To understand how you can interact with the API I built a short example, in this example I will:
- Pull image (I'm using light HTTP only to demonstrate an HTTP server)
- Create a container with this image with some configurations to map the ports
- Start container
- Wait some time to test the container
- Stop container
- Remove container
- Remove image
Using Docker-API GEM require 'docker' # For local tests Docker.url = 'unix:///var/run/docker.sock' # docker pull romainlecomte/lighthttpd-docker image = Docker::Image.create(fromImage: 'romainlecomte/lighthttpd-docker') puts "Image created! ID: #{image.id}" # docker create -p 8080:80 romainlecomte/lighthttpd-docker container = Docker::Container.create( 'Image': 'romainlecomte/lighthttpd-docker', 'ExposedPorts': { '80/tcp': {} }, 'HostConfig': { 'PortBindings': { '80/tcp': [{ 'HostPort': '8080' }] } } ) puts "Container created! ID: #{container.id}" # docker start <ID> container.start puts 'Container started and will stop after 10 seconds. Open http://localhost:8080 in your browser to see the HTTP Server.' # Wait some time 10.times do print '.' sleep 1 end # docker stop <ID> container.stop puts "\nContainer stopped" # docke rm <ID> container.remove puts 'Container removed' # docker rmi romainlecomte/lighthttpd-docker image.remove puts 'Image removed'
How cool is that? How many cool things can you do?